Building a Streamlit-Based Movie Recommender System Using Python
- Geoffrey Ogato
- Jun 20, 2024
- 4 min read
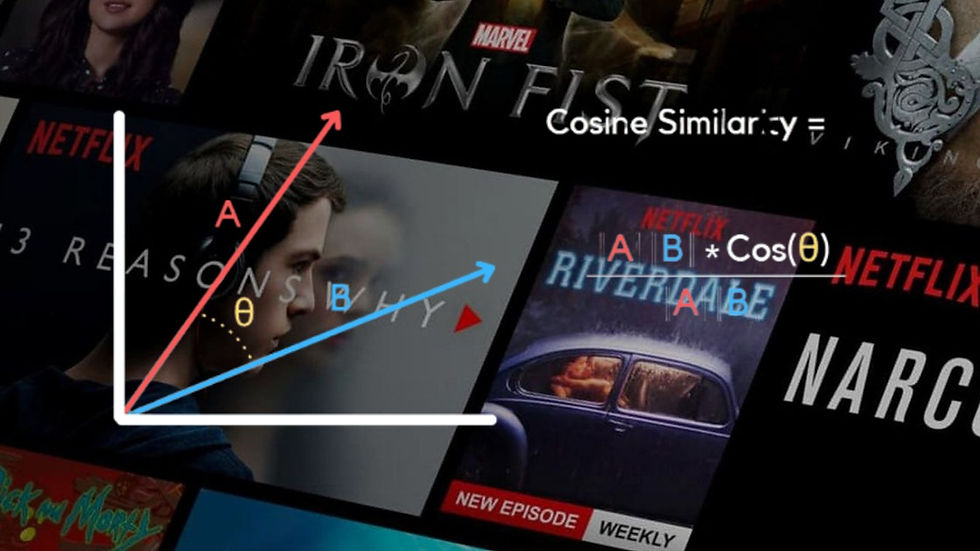
link to GitHub : https://github.com/Geoffrey-lab/Streamlit-Based-Movie-Recommender-System-Using-Python
Introduction
In today's digital world, recommendation systems play a crucial role in enhancing user experience across various platforms. Whether it's suggesting the next binge-worthy show on Netflix or recommending products on Amazon, these systems help users discover content tailored to their preferences. In this article, we'll delve into the creation of a Streamlit-based movie recommender system using Python. This project not only demonstrates the power of recommendation algorithms but also highlights the simplicity and elegance of using Streamlit for building interactive web applications.
1. Understanding Recommendation Systems
Recommendation systems are algorithms designed to suggest relevant items to users based on their preferences and behavior. There are several types of recommendation systems, including:
Collaborative Filtering: This method makes recommendations based on the preferences of similar users.
Content-Based Filtering: This approach suggests items similar to those a user has shown interest in.
Hybrid Systems: Combining multiple recommendation techniques to enhance accuracy and performance.
In our project, we use a hybrid approach by leveraging a similarity matrix that combines collaborative and content-based filtering.
2. Tools and Technologies
To build our movie recommender system, we utilize the following tools and technologies:
Streamlit: A powerful framework for building interactive web applications with Python.
FuzzyWuzzy: A library for string matching to improve the recommendation accuracy.
TMDB API: An external data source for fetching movie posters.
Python Libraries: Pandas for data manipulation, Pickle for saving and loading data, and Requests for handling HTTP requests.
3. Setting Up the Project
Before diving into the code, let's set up our project environment. Start by installing the necessary libraries:
pip install streamlit pandas requests fuzzywuzzy scikit-learn
4. Data Preparation
The foundation of our recommendation system lies in the data. We start by loading and exploring the dataset, followed by cleaning and preparing it for analysis. We'll create a similarity matrix to identify relationships between movies.
import pandas as pd
from sklearn.metrics.pairwise import cosine_similarity
movies = pd.read_csv("movies.csv")
# Clean and preprocess the data
similarity = cosine_similarity(movies_features)
# Save the similarity matrix
pickle.dump(similarity, open("similarity.pkl", "wb"))
5. Building the Streamlit App
Let's create a basic Streamlit app to display our movie recommender system. We'll start with setting up the structure and layout of the app.
import streamlit as st
import pickle
st.header("Movie Recommender System")
movies = pickle.load(open("movies_list.pkl", 'rb'))
similarity = pickle.load(open("similarity.pkl", 'rb'))
movies_list = movies['title'].values
selectvalue = st.selectbox("Select movie from dropdown", movies_list)
6. Fetching Movie Posters
To enhance the user experience, we'll fetch movie posters using the TMDB API. This adds a visual element to our recommendations.
import requests
def fetch_poster(movie_id):
url = f"https://api.themoviedb.org/3/movie/{movie_id}?api_key=YOUR_API_KEY&language=en-US"
data = requests.get(url).json()
poster_path = data['poster_path']
full_path = "https://image.tmdb.org/t/p/w500/" + poster_path
return full_path
7. Implementing the Recommendation Function
Using FuzzyWuzzy, we'll implement the recommendation function to provide accurate movie suggestions based on user input.
from fuzzywuzzy import process
def recommend(movie):
index = movies[movies['title'] == movie].index[0]
distances = sorted(list(enumerate(similarity[index])), reverse=True, key=lambda x: x[1])
recommend_movie = []
recommend_poster = []
for i in distances[1:6]:
movie_id = movies.iloc[i[0]].id
recommend_movie.append(movies.iloc[i[0]].title)
recommend_poster.append(fetch_poster(movie_id))
return recommend_movie, recommend_poster
8. Displaying Recommendations in Streamlit
We can now display the recommended movies and their posters in a visually appealing layout using Streamlit's column feature.
if st.button("Show Recommend"):
movie_name, movie_poster = recommend(selectvalue)
col1, col2, col3, col4, col5 = st.columns(5)
with col1:
st.text(movie_name[0])
st.image(movie_poster[0])
with col2:
st.text(movie_name[1])
st.image(movie_poster[1])
with col3:
st.text(movie_name[2])
st.image(movie_poster[2])
with col4:
st.text(movie_name[3])
st.image(movie_poster[3])
with col5:
st.text(movie_name[4])
st.image(movie_poster[4])
9. Deploying the Streamlit App
Deploying the app allows others to access and interact with your recommender system. Streamlit Sharing is a simple and effective way to deploy Streamlit apps.
Push your code to a GitHub repository.
Sign up on Streamlit Sharing and connect your GitHub account.
Deploy the app by selecting the repository and branch.
10. Conclusion
We've built a comprehensive movie recommender system using Streamlit and Python. This project showcases the power of recommendation algorithms and the ease of creating interactive web applications with Streamlit.
Potential Enhancements
Adding user authentication
Incorporating user ratings and feedback
Expanding the dataset for better recommendations
By building upon this project, you can create even more sophisticated and personalized recommendation systems.Embark on this journey to harness the power of recommendation systems and create applications that make a difference in the digital world. Happy coding!
Appendix
The full source code for this project is available on GitHub. Additional resources and references for further reading can be found in the repository.
4oy the app directly from the repository.
Full Source Code
import streamlit as st
import pickle
import requests
import streamlit.components.v1 as components
# Function to fetch movie posters using TMDB API
def fetch_poster(movie_id):
url = f"https://api.themoviedb.org/3/movie/{movie_id}?api_key=c7ec19ffdd3279641fb606d19ceb9bb1&language=en-US"
data = requests.get(url).json()
poster_path = data['poster_path']
full_path = "https://image.tmdb.org/t/p/w500/" + poster_path
return full_path
# Load movies and similarity matrix from Pickle files
movies = pickle.load(open("movies_list.pkl", 'rb'))
similarity = pickle.load(open("similarity.pkl", 'rb'))
movies_list = movies['title'].values
# Streamlit header
st.header("Movie Recommender System")
# Custom Streamlit component for image carousel
imageCarouselComponent = components.declare_component("image-carousel-component", path="frontend/public")
# Sample movie posters to display in the carousel
imageUrls = [
fetch_poster(1632),
fetch_poster(299536),
fetch_poster(17455),
fetch_poster(2830),
fetch_poster(429422),
fetch_poster(9722),
fetch_poster(13972),
fetch_poster(240),
fetch_poster(155),
fetch_poster(598),
fetch_poster(914),
fetch_poster(255709),
fetch_poster(572154)
]
# Display the image carousel
imageCarouselComponent(imageUrls=imageUrls, height=200)
# Dropdown for selecting a movie
selectvalue = st.selectbox("Select movie from dropdown", movies_list)
# Function to recommend movies based on the selected movie
def recommend(movie):
index = movies[movies['title'] == movie].index[0]
distances = sorted(list(enumerate(similarity[index])), reverse=True, key=lambda x: x[1])
recommend_movie = []
recommend_poster = []
for i in distances[1:6]:
movie_id = movies.iloc[i[0]].id
recommend_movie.append(movies.iloc[i[0]].title)
recommend_poster.append(fetch_poster(movie_id))
return recommend_movie, recommend_poster
# Button to display recommendations
if st.button("Show Recommend"):
movie_name, movie_poster = recommend(selectvalue)
col1, col2, col3, col4, col5 = st.columns(5)
with col1:
st.text(movie_name[0])
st.image(movie_poster[0])
with col2:
st.text(movie_name[1])
st.image(movie_poster[1])
with col3:
st.text(movie_name[2])
st.image(movie_poster[2])
with col4:
st.text(movie_name[3])
st.image(movie_poster[3])
with col5:
st.text(movie_name[4])
st.image(movie_poster[4])
# Footer
st.markdown('<p style="text-align: left;">Copyright © 2024; <b style="font-size:17px;">built by</b> <b style="font-size:25px;">Geoffrey Ogato</b></p>', unsafe_allow_html=True)
Explanation of the Code
By following this guide, you can build a fully functional movie recommender system and gain valuable insights into the fascinating world of recommendation algorithms. Happy coding!
Comments